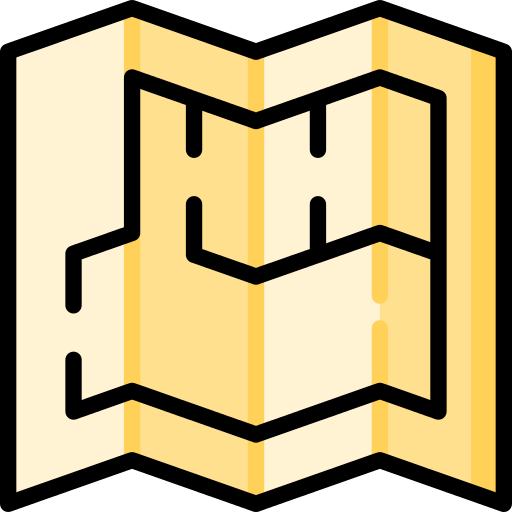
Dungeoneer
Procedurally generate beautiful 2d dungeons for your game.
https://lucianbuzzo.github.io/dungeoneer
This module is a tool for generating random dungeons as a two-dimensional array. It is largely based on the excellent work of Bob Nystrom and his game Hauberk, which you can read about here.
A demo of this module can be seen here https://lucianbuzzo.github.io/dungeoneer/
Installation
Install dungeoneer
by running:
$ npm install --save dungeoneer
Usage
const dungeoneer = const dungeon = dungeoneer
The build
method accepts width
and height
options that define the size of
the dungeon and will return a dungeon object. The smallest possible size for
a dungeon is 5 x 5. Dungeons are always an odd size due to the way walls and
floors are generated. If you supply even-sized dimensions, they will be rounded
up to the nearest odd number.
The shape of the dungeon object is defined below:
Seeding
A dungeon can be seeded using the seed
option. A dungeon created with a seed
and the same options can be repeatably created. Dungeons always return the seed
they were created with.
const dungeoneer = const dungeon = dungeoneer
License
The project is licensed under the MIT license.
The icon at the top of this file is provided by svgrepo.com and is licensed under Creative Commons BY 4.0.